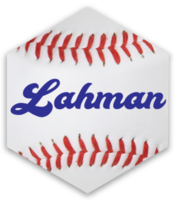
FieldingOF table
FieldingOF.Rd
Outfield position data: information about positions played in the outfield
Usage
data(FieldingOF)
Format
A data frame with 12380 observations on the following 6 variables.
playerID
Player ID code
yearID
Year
stint
player's stint (order of appearances within a season)
Glf
Games played in left field
Gcf
Games played in center field
Grf
Games played in right field
Source
Lahman, S. (2024) Lahman's Baseball Database, 1871-2023, 2024 version, http://www.seanlahman.com/
Examples
require("dplyr")
require("tidyr")
## Data set only goes through 1955
## Can get a more complete record from the Fielding data frame
## or from the Appearances data (see below)
## Output directly from the FieldingOF data
## Barry Bonds (no records: post-1955 player)
FieldingOF %>%
filter(playerID == "bondsba01")
#> [1] playerID yearID stint Glf Gcf Grf
#> <0 rows> (or 0-length row.names)
## Willie Mays (first few years)
FieldingOF %>%
filter(playerID == "mayswi01")
#> playerID yearID stint Glf Gcf Grf
#> 1 mayswi01 1951 1 0 121 0
#> 2 mayswi01 1952 1 0 34 0
#> 3 mayswi01 1954 1 0 151 0
#> 4 mayswi01 1955 1 0 152 0
## Ty Cobb (complete)
FieldingOF %>%
filter(playerID == "cobbty01")
#> playerID yearID stint Glf Gcf Grf
#> 1 cobbty01 1905 1 2 39 0
#> 2 cobbty01 1906 1 18 55 24
#> 3 cobbty01 1907 1 0 0 150
#> 4 cobbty01 1908 1 0 0 150
#> 5 cobbty01 1909 1 0 0 156
#> 6 cobbty01 1910 1 0 111 26
#> 7 cobbty01 1911 1 0 146 0
#> 8 cobbty01 1912 1 0 140 0
#> 9 cobbty01 1913 1 0 116 2
#> 10 cobbty01 1914 1 0 96 0
#> 11 cobbty01 1915 1 0 156 0
#> 12 cobbty01 1916 1 0 143 0
#> 13 cobbty01 1917 1 0 123 29
#> 14 cobbty01 1918 1 0 92 3
#> 15 cobbty01 1919 1 0 123 0
#> 16 cobbty01 1920 1 0 112 0
#> 17 cobbty01 1921 1 0 121 0
#> 18 cobbty01 1922 1 0 133 1
#> 19 cobbty01 1923 1 0 141 0
#> 20 cobbty01 1924 1 0 155 0
#> 21 cobbty01 1925 1 0 101 4
#> 22 cobbty01 1926 1 15 39 1
#> 23 cobbty01 1927 1 0 52 75
#> 24 cobbty01 1928 1 0 0 85
## One way to get OF game information from the Fielding data
## Note: OF games != sum(LF, CF, RF) because players can switch
## OF positions within a game. Players can also switch from
## other positions to outfield during a game. OF represents
## the number of games a player started in the outfield.
Fielding %>%
select(playerID, yearID, stint, POS, G) %>%
filter(POS %in% c("LF", "CF", "RF", "OF")) %>%
tidyr::spread(POS, G, fill = 0) %>%
filter(playerID == "trumbma01")
#> playerID yearID stint OF
#> 1 trumbma01 2010 1 1
#> 2 trumbma01 2011 1 11
#> 3 trumbma01 2012 1 97
#> 4 trumbma01 2013 1 27
#> 5 trumbma01 2014 1 41
#> 6 trumbma01 2015 1 42
#> 7 trumbma01 2015 2 46
#> 8 trumbma01 2016 1 96
#> 9 trumbma01 2017 1 31
#> 10 trumbma01 2018 1 19
## Another way is through the Appearances data (no stint).
## Provides a somewhat nicer table than the above.
## Mark Trumbo (active player)
Appearances %>%
select(playerID, yearID, G_lf, G_cf, G_rf, G_of) %>%
filter(playerID == "trumbma01")
#> playerID yearID G_lf G_cf G_rf G_of
#> 1 trumbma01 2010 0 0 1 1
#> 2 trumbma01 2011 1 0 10 11
#> 3 trumbma01 2012 66 0 35 97
#> 4 trumbma01 2013 8 0 19 27
#> 5 trumbma01 2014 41 0 0 41
#> 6 trumbma01 2015 0 0 42 42
#> 7 trumbma01 2015 13 0 34 46
#> 8 trumbma01 2016 1 0 95 96
#> 9 trumbma01 2017 0 0 31 31
#> 10 trumbma01 2018 0 0 19 19
#> 11 trumbma01 2019 0 0 0 0
## A slightly better format, perhaps
Appearances %>%
select(playerID, yearID, G_lf, G_cf, G_rf, G_of) %>%
rename(LF = G_lf, CF = G_cf, RF = G_rf, OF = G_of) %>%
filter(playerID == "trumbma01")
#> playerID yearID LF CF RF OF
#> 1 trumbma01 2010 0 0 1 1
#> 2 trumbma01 2011 1 0 10 11
#> 3 trumbma01 2012 66 0 35 97
#> 4 trumbma01 2013 8 0 19 27
#> 5 trumbma01 2014 41 0 0 41
#> 6 trumbma01 2015 0 0 42 42
#> 7 trumbma01 2015 13 0 34 46
#> 8 trumbma01 2016 1 0 95 96
#> 9 trumbma01 2017 0 0 31 31
#> 10 trumbma01 2018 0 0 19 19
#> 11 trumbma01 2019 0 0 0 0
## Willie Mays (1951-1973)
Appearances %>%
select(playerID, yearID, G_lf, G_cf, G_rf, G_of) %>%
filter(playerID == "mayswi01")
#> playerID yearID G_lf G_cf G_rf G_of
#> 1 mayswi01 1951 0 121 0 121
#> 2 mayswi01 1952 0 34 0 34
#> 3 mayswi01 1954 0 151 0 151
#> 4 mayswi01 1955 0 152 0 152
#> 5 mayswi01 1956 0 152 0 152
#> 6 mayswi01 1957 0 150 0 150
#> 7 mayswi01 1958 0 151 0 151
#> 8 mayswi01 1959 0 147 0 147
#> 9 mayswi01 1960 0 152 0 152
#> 10 mayswi01 1961 0 153 0 153
#> 11 mayswi01 1962 0 161 0 161
#> 12 mayswi01 1963 0 157 0 157
#> 13 mayswi01 1964 0 155 0 155
#> 14 mayswi01 1965 1 147 4 150
#> 15 mayswi01 1966 1 144 5 149
#> 16 mayswi01 1967 0 134 0 134
#> 17 mayswi01 1968 0 141 1 142
#> 18 mayswi01 1969 0 106 2 108
#> 19 mayswi01 1970 0 128 0 128
#> 20 mayswi01 1971 0 84 0 84
#> 21 mayswi01 1972 0 50 0 50
#> 22 mayswi01 1972 0 14 0 14
#> 23 mayswi01 1973 0 45 0 45
## Joe DiMaggio (1936-1951)
Appearances %>%
select(playerID, yearID, G_lf, G_cf, G_rf, G_of) %>%
filter(playerID == "dimagjo01")
#> playerID yearID G_lf G_cf G_rf G_of
#> 1 dimagjo01 1936 64 55 20 138
#> 2 dimagjo01 1937 0 150 0 150
#> 3 dimagjo01 1938 0 145 0 145
#> 4 dimagjo01 1939 0 116 0 116
#> 5 dimagjo01 1940 0 130 0 130
#> 6 dimagjo01 1941 0 139 0 139
#> 7 dimagjo01 1942 0 154 0 154
#> 8 dimagjo01 1946 3 128 0 131
#> 9 dimagjo01 1947 0 139 0 139
#> 10 dimagjo01 1948 0 152 0 152
#> 11 dimagjo01 1949 0 76 0 76
#> 12 dimagjo01 1950 0 137 0 137
#> 13 dimagjo01 1951 0 113 0 113