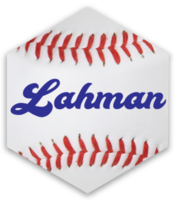
BattingPost table
BattingPost.Rd
Post season batting statistics
Usage
data(BattingPost)
Format
A data frame with 16857 observations on the following 22 variables.
yearID
Year
round
Level of playoffs
playerID
Player ID code
teamID
Team
lgID
League; a factor with levels
AA
AL
NL
G
Games
AB
At Bats
R
Runs
H
Hits
X2B
Doubles
X3B
Triples
HR
Homeruns
RBI
Runs Batted In
SB
Stolen Bases
CS
Caught stealing
BB
Base on Balls
SO
Strikeouts
IBB
Intentional walks
HBP
Hit by pitch
SH
Sacrifices
SF
Sacrifice flies
GIDP
Grounded into double plays
Source
Lahman, S. (2024) Lahman's Baseball Database, 1871-2023, 2024 version, http://www.seanlahman.com/
Examples
# Post-season batting data
# Requires care since intra-league playoffs have evolved since 1969
# Simplest case: World Series
require("dplyr")
# Create a sub-data frame for modern World Series play
ws <- BattingPost %>%
filter(round == "WS" & yearID >= 1903) %>%
mutate(BA = 0 + (AB > 0) * round(H/AB, 3),
TB = H + X2B + 2 * X3B + 3 * HR,
SA = 0 + (AB > 0) * round(TB/AB, 3),
PA = AB + BB + IBB + HBP + SH + SF,
OB = H + BB + IBB + HBP,
OBP = 0 + (AB > 0) * round(OB/PA, 3) )
# Players with most appearances in the WS:
ws %>% group_by(playerID) %>%
summarise(appearances = n()) %>%
arrange(desc(appearances)) %>%
head(., 10)
#> # A tibble: 10 × 2
#> playerID appearances
#> <chr> <int>
#> 1 berrayo01 14
#> 2 mantlmi01 12
#> 3 fordwh01 11
#> 4 dimagjo01 10
#> 5 howarel01 10
#> 6 ruthba01 10
#> 7 bauerha01 9
#> 8 rizzuph01 9
#> 9 dickebi01 8
#> 10 friscfr01 8
# Non-Yankees with most WS appearances
ws %>% filter(teamID != "NYA") %>%
group_by(playerID) %>%
summarise(appearances = n()) %>%
arrange(desc(appearances)) %>%
head(., 10)
#> # A tibble: 10 × 2
#> playerID appearances
#> <chr> <int>
#> 1 friscfr01 8
#> 2 furilca01 7
#> 3 gilliji01 7
#> 4 hodgegi01 7
#> 5 reesepe01 7
#> 6 collied01 6
#> 7 palmeji01 6
#> 8 robinja02 6
#> 9 rosepe01 6
#> 10 snidedu01 6
# Top ten single WS batting averages ( >= 10 AB )
ws %>% filter(AB > 10) %>%
arrange(desc(BA)) %>%
head(., 10)
#> yearID round playerID teamID lgID G AB R H X2B X3B HR RBI SB CS BB SO IBB
#> 1 1990 WS hatchbi01 CIN NL 4 12 6 9 4 1 0 2 0 1 2 0 1
#> 2 2013 WS ortizda01 BOS AL 6 16 7 11 2 0 2 6 0 0 8 1 4
#> 3 1928 WS ruthba01 NYA AL 4 16 9 10 3 0 3 4 0 0 1 2 0
#> 4 2009 WS matsuhi01 NYA AL 6 13 3 8 1 0 3 8 0 0 1 3 0
#> 5 2001 WS bautida01 ARI NL 5 12 1 7 2 0 0 7 0 0 1 1 0
#> 6 1990 WS saboch01 CIN NL 4 16 2 9 1 0 2 5 0 1 2 2 0
#> 7 1914 WS gowdyha01 BSN NL 4 11 3 6 3 1 1 3 1 0 5 1 0
#> 8 1928 WS gehrilo01 NYA AL 4 11 5 6 1 0 4 9 0 0 6 0 0
#> 9 1999 WS boonebr01 ATL NL 4 13 1 7 4 0 0 3 0 1 1 3 0
#> 10 1976 WS benchjo01 CIN NL 4 15 4 8 1 1 2 6 0 0 0 1 0
#> HBP SH SF GIDP BA TB SA PA OB OBP
#> 1 1 0 0 1 0.750 15 1.250 16 13 0.812
#> 2 0 0 1 0 0.688 19 1.188 29 23 0.793
#> 3 0 0 0 1 0.625 22 1.375 17 11 0.647
#> 4 0 0 0 0 0.615 18 1.385 14 9 0.643
#> 5 0 0 0 0 0.583 9 0.750 13 8 0.615
#> 6 0 0 0 0 0.562 16 1.000 18 11 0.611
#> 7 0 0 0 0 0.545 14 1.273 16 11 0.688
#> 8 0 0 0 0 0.545 19 1.727 17 12 0.706
#> 9 0 0 0 0 0.538 11 0.846 14 8 0.571
#> 10 0 0 0 2 0.533 17 1.133 15 8 0.533
# Top ten slugging averages in a single WS
ws %>% filter(AB > 10) %>%
arrange(desc(SA)) %>%
head(., 10)
#> yearID round playerID teamID lgID G AB R H X2B X3B HR RBI SB CS BB SO IBB
#> 1 1928 WS gehrilo01 NYA AL 4 11 5 6 1 0 4 9 0 0 6 0 0
#> 2 2009 WS matsuhi01 NYA AL 6 13 3 8 1 0 3 8 0 0 1 3 0
#> 3 1928 WS ruthba01 NYA AL 4 16 9 10 3 0 3 4 0 0 1 2 0
#> 4 2002 WS bondsba01 SFN NL 7 17 8 8 2 0 4 6 0 0 13 3 7
#> 5 1914 WS gowdyha01 BSN NL 4 11 3 6 3 1 1 3 1 0 5 1 0
#> 6 1977 WS jacksre01 NYA AL 6 20 10 9 1 0 5 8 0 0 3 4 0
#> 7 1990 WS hatchbi01 CIN NL 4 12 6 9 4 1 0 2 0 1 2 0 1
#> 8 1939 WS kellech01 NYA AL 4 16 8 7 1 1 3 6 0 0 1 2 0
#> 9 2013 WS ortizda01 BOS AL 6 16 7 11 2 0 2 6 0 0 8 1 4
#> 10 2018 WS pearcst01 BOS AL 5 12 5 4 1 0 3 8 0 0 4 0 0
#> HBP SH SF GIDP BA TB SA PA OB OBP
#> 1 0 0 0 0 0.545 19 1.727 17 12 0.706
#> 2 0 0 0 0 0.615 18 1.385 14 9 0.643
#> 3 0 0 0 1 0.625 22 1.375 17 11 0.647
#> 4 0 0 0 0 0.471 22 1.294 37 28 0.757
#> 5 0 0 0 0 0.545 14 1.273 16 11 0.688
#> 6 1 0 0 1 0.450 25 1.250 24 13 0.542
#> 7 1 0 0 1 0.750 15 1.250 16 13 0.812
#> 8 0 0 0 0 0.438 19 1.188 17 8 0.471
#> 9 0 0 1 0 0.688 19 1.188 29 23 0.793
#> 10 0 0 0 0 0.333 14 1.167 16 8 0.500
# Hitting stats for the 1946 St. Louis Cardinals, ordered by BA
ws %>%
filter(teamID == "SLN" & yearID == 1946) %>%
arrange(desc(BA))
#> yearID round playerID teamID lgID G AB R H X2B X3B HR RBI SB CS BB SO IBB
#> 1 1946 WS ricede01 SLN NL 3 6 2 3 1 0 0 0 0 0 2 0 2
#> 2 1946 WS walkeha01 SLN NL 7 17 3 7 2 0 0 6 0 1 4 2 2
#> 3 1946 WS dicksmu01 SLN NL 2 5 1 2 2 0 0 1 0 0 0 1 0
#> 4 1946 WS slaugen01 SLN NL 7 25 5 8 1 1 1 2 1 0 4 3 2
#> 5 1946 WS garagjo01 SLN NL 5 19 2 6 2 0 0 4 0 0 0 3 0
#> 6 1946 WS kurowwh01 SLN NL 7 27 5 8 3 0 0 2 0 0 0 3 0
#> 7 1946 WS dusaker01 SLN NL 4 4 0 1 1 0 0 0 0 0 2 2 0
#> 8 1946 WS marioma01 SLN NL 7 24 1 6 2 0 0 4 0 0 1 1 1
#> 9 1946 WS mungere01 SLN NL 1 4 0 1 0 0 0 0 0 0 0 2 0
#> 10 1946 WS schoere01 SLN NL 7 30 3 7 1 0 0 1 1 1 0 2 0
#> 11 1946 WS musiast01 SLN NL 7 27 3 6 4 1 0 4 1 0 4 2 0
#> 12 1946 WS moorete01 SLN NL 7 27 1 4 0 0 0 2 0 0 2 6 0
#> 13 1946 WS brechha01 SLN NL 3 8 2 1 0 0 0 1 0 0 0 1 0
#> 14 1946 WS brazlal01 SLN NL 1 2 0 0 0 0 0 0 0 0 0 0 0
#> 15 1946 WS jonesni01 SLN NL 1 1 0 0 0 0 0 0 0 0 0 1 0
#> 16 1946 WS polleho01 SLN NL 2 4 0 0 0 0 0 0 0 0 0 1 0
#> 17 1946 WS sisledi01 SLN NL 2 2 0 0 0 0 0 0 0 0 0 0 0
#> 18 1946 WS beazljo01 SLN NL 1 0 0 0 0 0 0 0 0 0 0 0 0
#> 19 1946 WS wilkste01 SLN NL 1 0 0 0 0 0 0 0 0 0 0 0 0
#> HBP SH SF GIDP BA TB SA PA OB OBP
#> 1 0 0 0 0 0.500 4 0.667 10 7 0.700
#> 2 0 1 0 0 0.412 9 0.529 24 13 0.542
#> 3 0 0 0 0 0.400 4 0.800 5 2 0.400
#> 4 1 0 0 0 0.320 14 0.560 32 15 0.469
#> 5 0 0 0 0 0.316 8 0.421 19 6 0.316
#> 6 1 0 0 1 0.296 11 0.407 28 9 0.321
#> 7 0 0 0 0 0.250 2 0.500 6 3 0.500
#> 8 0 3 0 1 0.250 8 0.333 29 8 0.276
#> 9 0 1 0 0 0.250 1 0.250 5 1 0.200
#> 10 0 1 0 0 0.233 8 0.267 31 7 0.226
#> 11 0 0 0 0 0.222 12 0.444 31 10 0.323
#> 12 0 2 0 0 0.148 4 0.148 31 6 0.194
#> 13 0 0 0 0 0.125 1 0.125 8 1 0.125
#> 14 0 0 0 0 0.000 0 0.000 2 0 0.000
#> 15 0 0 0 0 0.000 0 0.000 1 0 0.000
#> 16 0 0 0 0 0.000 0 0.000 4 0 0.000
#> 17 0 0 0 0 0.000 0 0.000 2 0 0.000
#> 18 0 0 0 0 NaN 0 NaN 0 0 NaN
#> 19 0 0 0 0 NaN 0 NaN 0 0 NaN
# Babe Ruth's WS profile
ws %>%
filter(playerID == "ruthba01") %>%
arrange(yearID)
#> yearID round playerID teamID lgID G AB R H X2B X3B HR RBI SB CS BB SO IBB
#> 1 1915 WS ruthba01 BOS AL 1 1 0 0 0 0 0 0 0 0 0 0 0
#> 2 1916 WS ruthba01 BOS AL 1 5 0 0 0 0 0 1 0 0 0 2 0
#> 3 1918 WS ruthba01 BOS AL 3 5 0 1 0 1 0 2 0 0 0 2 0
#> 4 1921 WS ruthba01 NYA AL 6 16 3 5 0 0 1 4 2 1 5 8 0
#> 5 1922 WS ruthba01 NYA AL 5 17 1 2 1 0 0 1 0 1 2 3 0
#> 6 1923 WS ruthba01 NYA AL 6 19 8 7 1 1 3 3 0 0 8 6 0
#> 7 1926 WS ruthba01 NYA AL 7 20 6 6 0 0 4 5 1 1 11 2 1
#> 8 1927 WS ruthba01 NYA AL 4 15 4 6 0 0 2 7 1 0 2 2 1
#> 9 1928 WS ruthba01 NYA AL 4 16 9 10 3 0 3 4 0 0 1 2 0
#> 10 1932 WS ruthba01 NYA AL 4 15 6 5 0 0 2 6 0 0 4 3 0
#> HBP SH SF GIDP BA TB SA PA OB OBP
#> 1 0 0 0 0 0.000 0 0.000 1 0 0.000
#> 2 0 0 0 0 0.000 0 0.000 5 0 0.000
#> 3 0 1 0 0 0.200 3 0.600 6 1 0.167
#> 4 0 0 0 0 0.312 8 0.500 21 10 0.476
#> 5 1 1 0 0 0.118 3 0.176 21 5 0.238
#> 6 0 0 0 0 0.368 19 1.000 27 15 0.556
#> 7 0 0 0 0 0.300 18 0.900 32 18 0.562
#> 8 0 0 1 1 0.400 12 0.800 19 9 0.474
#> 9 0 0 0 1 0.625 22 1.375 17 11 0.647
#> 10 1 0 0 1 0.333 11 0.733 20 10 0.500